In my last post I showed how I do basic project configuration. It's time to do the standard "Hello World" and make sure things work.
Recall that in that post we created an empty "HelloWorld" project.
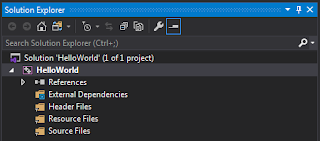 |
Empty HelloWorld Project |
In the view above, you see what I call a "filter view". This does not actually represent our files on disk but, rather, the types of files you have (source, headers, etc). If we started adding source and header files now these would be dropped in among the various other files that Visual Studio uses to manage the entire solution. That wouldn't be ideal at all for large projects so I want to set up folders that will contain my source and include files. To do this I switch from the default filter view to the files view. If you click that icon that looks like a stack of papers you'll switch to file view.
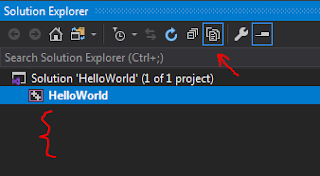 |
Stack of papers |
Notice that the file view is empty. We need to create two folders. We might need more, but these two will allow us to start simple. Right click the project "HelloWorld" and Add a New Folder named "src". Repeat this for "include".
If you look in Windows Explorer and find your project, you'll see the new folders. Inside each we will place any needed source or header files. Neat and tidy.
Right click the "src" folder and Add a New Item. Select C++ and click Add. I kept the default "Source.cpp" filename since this is just a simple Hello World test. Also note that the source file location is set to our "src" folder.
In our new C++ file we can enter the ubiquitous Hello World code!
#include <iostream>
int main(int argc, char* argv[])
{
std::cout << "Hello world!" << std::endl;
return 0;
}
(Blogger doesn't have a codeblock tool so that looks pretty ugly.)
Hit F5 (to run with debugging) or Cntl-F5 (to run without debugging).
The program will compile and link. Output will be in the proper output directory. All intermediate files will be in the Intermediate Directory. Neat and clean.
If you get goofy errors, be sure to check that your platform settings are appropriate. My empty projects seem to always start with the x86 platform by default. Every so often I'll get an error (usually during linking) and realize that I forgot to set the platform. This sort of thing would be rather loud if we were linking an x64 program against x86 libraries
Below you'll see that compile and link was successful and that we can run HelloWorld.exe successfully.
This is just a simple Hello World but I hope this is helpful to anyone just getting their feet wet with Visual Studio. As always, I welcome constructive criticism. It helps me learn and get better at this stuff.